Crypto Genius/Amm
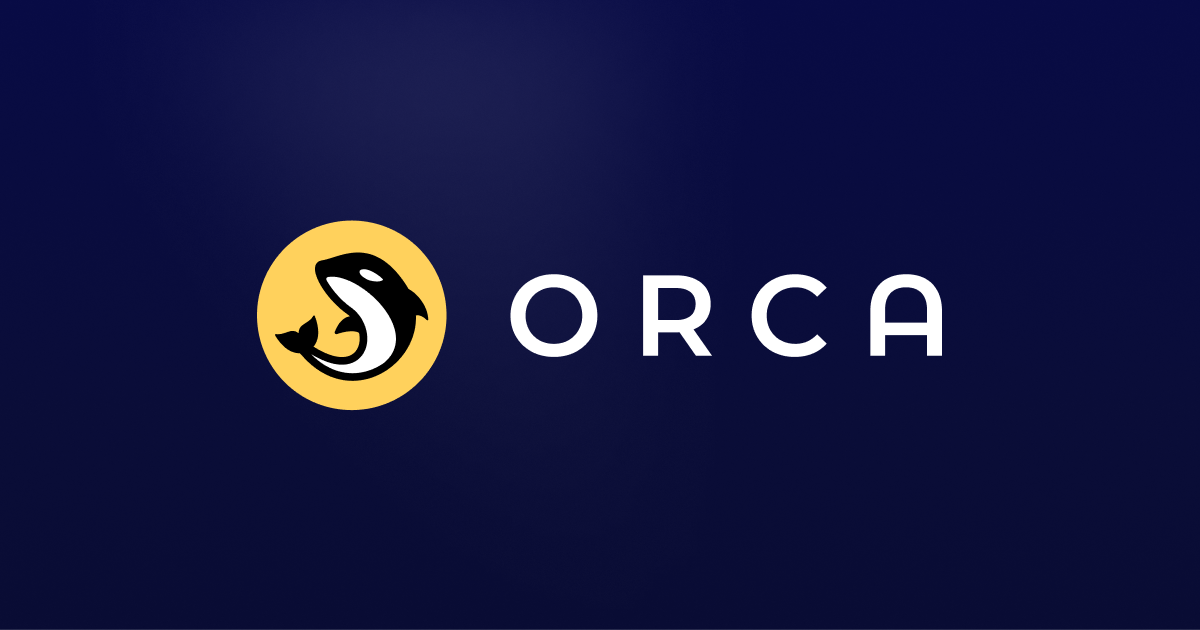
This is my chat with Anthropic to prepare for an interview with an AMM.
Simple AMM swap
pub fn calculate_swap_output(
input_amount: u64,
input_reserve: u64,
output_reserve: u64,
fee_numerator: u64,
fee_denominator: u64,
) -> Result<u64, String> {
if input_amount == 0 || input_reserve == 0 || output_reserve == 0 {
return Err("Invalid input parameters".to_string());
}
// Calculate fee
let input_amount_with_fee = input_amount.checked_mul(fee_denominator.checked_sub(fee_numerator).ok_or("Overflow")?).ok_or("Overflow")?
.checked_div(fee_denominator).ok_or("Division by zero")?;
// Calculate output based on constant product formula: x * y = k
let numerator = input_amount_with_fee.checked_mul(output_reserve).ok_or("Overflow")?;
let denominator = input_reserve.checked_add(input_amount_with_fee).ok_or("Overflow")?;
numerator.checked_div(denominator).ok_or("Division by zero".to_string())
}
AMM Knowledge:
- Constant product formula (x * y = k) and its implications
- Concentrated liquidity (CLAMMs) vs traditional AMMs
- Impermanent loss and how it affects liquidity providers
- Price impact and slippage in AMM trades
- Orca-Specific Knowledge:
- Orca's Whirlpools
- Orca's position as a leading Solana DEX
- Market making strategies on Orca
- Comparison with other Solana DEXs (Jupiter, Raydium)
- Solana Ecosystem Understanding:
- Advantages of Solana for DeFi (speed, cost, composability)
- Challenges in the Solana ecosystem and how Orca addresses them
- Recent developments in Solana DeFi
1. Rust Backend Systems
- Asynchronous programming in Rust with Tokio
- Error handling patterns in Rust
- Memory safety and performance considerations
- Connecting Rust servers to Solana RPC nodes
Example question: How would you design a high-performance event processor for Solana transactions?
2. Solana Program Development
- Explain the account model in Solana
- Discuss program derived addresses (PDAs)
- Handle cross-program invocations (CPIs)
- Implement secure Anchor programs
Example question: Design a simple liquidity pool program that handles deposits, withdrawals, and swaps.
3. Data Processing and Infrastructure
- Database design for blockchain data
- Scaling considerations for high-throughput systems
- AWS infrastructure for DeFi applications
- Data pipeline architecture
Example question: How would you design a system to process and index all Orca pool events with minimal latency?
Brainstorming ideas:
- How would you design a backend service to monitor Solana liquidity pools in real-time?
- Explain how you would handle concurrency in a Rust server processing thousands of blockchain events per second.
- How would you implement a price impact calculation for an AMM swap?
- Describe your approach to error handling in Rust, especially when dealing with blockchain transactions that might fail.
- How would you design a database schema to track historical liquidity and volume for thousands of trading pairs?
Chat continuation...
Answer this question: "How would you design a backend service to monitor Solana liquidity pools in real-time?"
I'll provide a detailed answer for the question on designing a real-time Solana liquidity pool monitoring service.
To design a backend service that monitors Solana liquidity pools in real-time, I'd create a system with these key components:
Data Ingestion Layer
- Geyser Plugin Integration
- I'd leverage Solana's Geyser plugin which provides real-time account updates directly from the validator
- Alternatively, I'd use Yellowstone gRPC (as mentioned in Orca's stack) for efficient streaming of on-chain data
- Transaction Parser
- Implement custom parsers for pool-specific instructions (deposits, withdrawals, swaps)
- Focus on decoding relevant Orca CLAMM and Whirlpool transactions
Processing Layer
- Asynchronous Event Processing
- Use Tokio for asynchronous Rust to handle high-throughput event streams
- Implement backpressure mechanisms to handle traffic spikes
- Process events through a priority queue based on transaction age
- State Management
- Maintain an in-memory state of all active pools
- Use concurrent data structures like
Arc<RwLock<T>>
for thread-safe access - Implement change detection to identify significant liquidity movements
Storage Layer
- Time-Series Database
- Use TimescaleDB (part of Orca's stack) for efficient time-series data storage
- Implement hypertables for fast range queries on historical data
- Design efficient partitioning strategies based on pool activity patterns
- Data Aggregation
- Create pre-aggregated views for common queries (hourly, daily summaries)
- Implement materialized views for expensive calculations
API Layer
- GraphQL/REST Endpoints
- Provide flexible query capabilities for frontend applications
- Implement WebSocket connections for real-time data streaming
- Design rate limiting based on usage patterns
- Query Optimization
- Cache frequently accessed pool states
- Implement query result pagination
Monitoring & Reliability
- Fault Tolerance
- Deploy across multiple availability zones
- Implement circuit breakers for RPC node connections
- Design replay capability from missed blocks
- Performance Monitoring
- Track event processing latency
- Monitor database query performance
- Set up alerting for anomalies in pool behavior
Scaling Considerations
- Horizontal Scaling
- Shard pools across multiple processing instances
- Scale read replicas for database queries
- Implement load balancing for API requests
- Optimization
- Use compiled Rust for performance-critical path
- Implement batch processing where appropriate
This architecture provides real-time monitoring of Solana liquidity pools with high reliability and performance, while maintaining the ability to scale as the number of pools and transaction volume grows.
Chat continuation...
Please delve deeper for the following concepts: Implement backpressure mechanisms to handle traffic spikes Process events through a priority queue based on transaction age Implement change detection to identify significant liquidity movements Why would I store the anything using TimescaleDB (part of Orca's stack) for efficient time-series data storage, if it's just about monitoring? What are hypertables for fast range queries on historical data Delve deeper on "Design rate limiting based on usage patterns" Delve deeper on "Implement circuit breakers for RPC node connections"
Let me provide deeper explanations for each of these concepts:
Backpressure Mechanisms to Handle Traffic Spikes
Backpressure is crucial when processing real-time blockchain data because Solana can produce sudden transaction spikes (30k-50k TPS during peak periods):
// Example implementation using Tokio channels with bounded capacity
let (tx, rx) = tokio::sync::mpsc::channel::<SolanaEvent>(10_000); // Fixed buffer size
// Producer with backpressure
async fn produce_events(tx: mpsc::Sender<SolanaEvent>, event_stream: EventStream) {
for event in event_stream {
// Will wait if the channel is full, creating backpressure
if let Err(e) = tx.send(event).await {
log::error!("Channel full, implementing backpressure: {}", e);
// Introduce exponential backoff here
tokio::time::sleep(Duration::from_millis(100)).await;
}
}
}
// Consumer that processes at its own pace
async fn process_events(mut rx: mpsc::Receiver<SolanaEvent>) {
while let Some(event) = rx.recv().await {
// Process event at sustainable rate
process_pool_event(event).await;
}
}
This approach prevents memory exhaustion during traffic spikes by having producers slow down when consumers can't keep up.
Priority Queue Based on Transaction Age
When monitoring liquidity pools, newer transactions may need to wait for older ones to maintain causality:
#[derive(Eq, PartialEq)]
struct PrioritizedEvent {
slot: u64,
tx_index: u64,
event: SolanaEvent,
}
// Custom ordering implementation
impl Ord for PrioritizedEvent {
fn cmp(&self, other: &Self) -> std::cmp::Ordering {
// First by slot number (block height)
match self.slot.cmp(&other.slot) {
std::cmp::Ordering::Equal => {
// Then by transaction index within the block
self.tx_index.cmp(&other.tx_index)
}
other => other,
}
}
}
// Priority queue implementation
let mut event_queue = BinaryHeap::new();
// Process in causal order
while let Some(event) = event_queue.pop() {
update_pool_state(event.event).await;
}
This ensures that pool states are updated in the correct sequence, preventing state corruption from out-of-order processing.
Change Detection for Significant Liquidity Movements
Not all pool updates are equally important. Detecting meaningful changes:
struct PoolState {
liquidity: u128,
token_a_amount: u64,
token_b_amount: u64,
last_update: SystemTime,
}
fn detect_significant_changes(old_state: &PoolState, new_state: &PoolState) -> bool {
// Calculate percentage changes
let liquidity_change_pct = percentage_change(old_state.liquidity, new_state.liquidity);
let price_impact = calculate_price_impact(
old_state.token_a_amount, old_state.token_b_amount,
new_state.token_a_amount, new_state.token_b_amount
);
// Detect significant movements using adaptive thresholds
let time_since_last_update = new_state.last_update.duration_since(old_state.last_update).unwrap();
let threshold = adaptive_threshold(time_since_last_update);
// Return true if any metric exceeds threshold
liquidity_change_pct > threshold || price_impact > threshold
}
This approach filters noise and focuses on meaningful state changes, reducing downstream processing.
Why Use TimescaleDB for Monitoring?
While "monitoring" might suggest just real-time alerting, there are several reasons to use TimescaleDB:
- Historical Analysis: Understanding pool behavior requires historical context (e.g., "how has this pool's liquidity changed over the last 24 hours?")
- Anomaly Detection: You need historical data to establish baselines for what constitutes "unusual" activity
- Performance Optimization: Query patterns for monitoring liquidity are time-series by nature (e.g., "show me liquidity changes in 5-minute intervals")
- Compliance and Auditing: Maintaining an immutable record of all pool activity for potential regulatory requirements
TimescaleDB is particularly suited because it optimizes for time-series data while providing the full power of PostgreSQL.
Hypertables for Fast Range Queries
Hypertables are TimescaleDB's way of automatically partitioning time-series data:
sqlCopy-- Create a regular table
CREATE TABLE pool_events (
time TIMESTAMPTZ NOT NULL,
pool_id TEXT NOT NULL,
liquidity NUMERIC,
price NUMERIC,
volume_24h NUMERIC
);
-- Convert to hypertable partitioned by time
SELECT create_hypertable('pool_events', 'time', chunk_time_interval => INTERVAL '1 day');
Benefits:
- Chunk Pruning: When querying a specific time range, TimescaleDB only scans relevant chunks
- Parallel Query Execution: Each chunk can be processed in parallel
- Efficient Data Retention: Older chunks can be compressed or moved to slower storage
This drastically improves query performance for range-based queries like "show me all pool activity from the last week."
Rate Limiting Based on Usage Patterns
Advanced rate limiting should adapt to usage patterns rather than applying fixed limits:
struct AdaptiveRateLimiter {
user_limits: HashMap<UserId, RateLimit>,
usage_history: HashMap<UserId, VecDeque<Instant>>,
pattern_analyzer: PatternAnalyzer,
}
impl AdaptiveRateLimiter {
fn should_allow_request(&mut self, user_id: UserId, endpoint: &str) -> bool {
// Retrieve user history
let history = self.usage_history.entry(user_id).or_default();
history.push_back(Instant::now());
// Clean old entries
while let Some(time) = history.front() {
if time.elapsed() > Duration::from_secs(3600) {
history.pop_front();
} else {
break;
}
}
// Analyze pattern for this endpoint
let pattern = self.pattern_analyzer.analyze_usage(endpoint, history);
// Apply different strategies based on pattern
match pattern {
UsagePattern::Bursty => self.apply_token_bucket(user_id, endpoint),
UsagePattern::Steady => self.apply_fixed_window(user_id, endpoint),
UsagePattern::Periodic => self.apply_sliding_window(user_id, endpoint),
}
}
}
This allows legitimate bursts of activity (like reacting to a market event) while still preventing abuse.
Circuit Breakers for RPC Node Connections
RPC nodes can fail or become unresponsive, especially during network congestion:
rustCopystruct RpcConnectionPool {
connections: Vec<RpcConnection>,
health_metrics: HashMap<RpcNodeId, HealthMetrics>,
circuit_breaker_config: CircuitBreakerConfig,
}
impl RpcConnectionPool {
async fn execute_rpc<T>(&mut self, request: RpcRequest) -> Result<T, RpcError> {
// Select best connection based on health
let connection = self.select_healthy_connection()?;
// Attempt to execute the request
match timeout(Duration::from_secs(5), connection.execute(request)).await {
Ok(result) => {
// Update success metrics
self.record_success(&connection.id);
result },
Err(_) => {
// Timeout occurred - update failure metrics
self.record_failure(&connection.id);
// Trip circuit breaker if error threshold exceeded
if self.should_trip_circuit_breaker(&connection.id) {
self.trip_circuit_breaker(&connection.id);
}
// Retry with different connection
self.retry_with_fallback(request).await
}
}
}
fn should_trip_circuit_breaker(&self, node_id: &RpcNodeId) -> bool {
let metrics = &self.health_metrics[node_id];
// Check error rate
let error_rate = metrics.errors_last_minute as f64 / (metrics.errors_last_minute + metrics.successes_last_minute) as f64;
// Trip if error rate too high or response times too slow
latency_threshold
error_rate > self.circuit_breaker_config.error_threshold ||
metrics.avg_response_time > self.circuit_breaker_config. }
fn trip_circuit_breaker(&mut self, node_id: &RpcNodeId) {
// Mark connection as unavailable for configured time period
self.health_metrics.get_mut(node_id).unwrap().available = false;
// Schedule half-open state after timeout
let node_id_clone = node_id.clone();
let pool = self.clone();
tokio::spawn(async move {
tokio::time::sleep(pool.circuit_breaker_config.reset_timeout).await;
pool.half_open_circuit(&node_id_clone);
});
}
}
This approach:
- Monitors RPC node health metrics in real-time
- Removes unhealthy nodes automatically when they exceed error thresholds
- Gradually reintroduces nodes after a cooling-off period
- Prevents cascading failures when the Solana network is under stress
These deeper implementations would create a resilient, high-performance system capable of reliably monitoring Solana liquidity pools even during extreme network conditions or traffic spikes.
Question
Answer this question "Explain how you would handle concurrency in a Rust server processing thousands of blockchain events per second."
To handle concurrency in a Rust server processing thousands of blockchain events per second, I'd implement a multi-layered approach leveraging Rust's safety guarantees and async runtime capabilities:
Tokio-Based Async Runtime Architecture
I'd build the system on Tokio's multi-threaded runtime to efficiently utilize all CPU cores:
rustCopy#[tokio::main(flavor = "multi_thread", worker_threads = 16)]
async fn main() {
// Configure the runtime with a thread pool sized to the system
let runtime = tokio::runtime::Builder::new_multi_thread()
.worker_threads(num_cpus::get())
.enable_io()
.enable_time()
.build()
.unwrap();
// Run the server within this runtime
runtime.block_on(run_server());
}
Event Ingestion and Sharding
To handle high throughput, I'd implement a sharded approach to event processing:
async fn run_server() {
// Create multiple event processors, each handling a subset of pools
let processor_count = num_cpus::get() * 2; // 2x oversubscription for IO-bound work
let mut processors = Vec::with_capacity(processor_count);
for i in 0..processor_count {
let processor = EventProcessor::new(i, processor_count);
processors.push(tokio::spawn(processor.run()));
}
// Set up Solana event stream
let event_stream = setup_solana_event_stream().await;
// Process incoming events
while let Some(event) = event_stream.next().await {
// Determine which processor should handle this event based on pool ID
let pool_id = extract_pool_id(&event);
let processor_idx = determine_shard(pool_id, processor_count);
// Send to appropriate processor
processors[processor_idx].send(event).await;
}
}
fn determine_shard(pool_id: &str, shard_count: usize) -> usize {
// Consistent hashing to ensure the same pool always goes to the same processor
let hash = seahash::hash(pool_id.as_bytes());
(hash % shard_count as u64) as usize
}
Lock-Free Concurrency with Message Passing
For communication between components, I'd use channels rather than shared memory:
rustCopystruct EventProcessor {
id: usize,
receiver: mpsc::Receiver<BlockchainEvent>,
pool_states: HashMap<String, Arc<RwLock<PoolState>>>,
}
impl EventProcessor {
async fn run(&mut self) {
while let Some(event) = self.receiver.recv().await {
// Process event without blocking other processors
self.process_event(event).await;
}
}
async fn process_event(&mut self, event: BlockchainEvent) {
pool_states
// Get the pool state with minimal locking
let pool_id = extract_pool_id(&event);
let pool_state = self. .entry(pool_id.to_string())
.or_insert_with(|| Arc::new(RwLock::new(PoolState::default())));
// Use fine-grained locking
{
// Prefer read locks when possible
let state = pool_state.read().await;
if !needs_update(&state, &event) {
return; // Early return without acquiring write lock
}
}
// Only acquire write lock when necessary
let mut state = pool_state.write().await;
update_pool_state(&mut state, &event);
}
}
Work Stealing for Load Balancing
To handle uneven workloads across shards:
rustCopystruct WorkStealingScheduler {
local_queue: VecDeque<Task>,
global_queue: Arc<Mutex<VecDeque<Task>>>,
other_workers: Vec<Worker>,
}
impl WorkStealingScheduler {
async fn execute(&mut self) {
loop {
// First try local queue
if let Some(task) = self.local_queue.pop_front() {
task.execute().await;
continue;
}
// Then try global queue
if let Some(task) = self.global_queue.lock().await.pop_front() {
task.execute().await;
continue;
}
// Finally try stealing from other workers
for worker in &self.other_workers {
if let Some(stolen_task) = worker.steal_task().await {
stolen_task.execute().await;
break;
}
}
}
}
}
State Management with MVCC Pattern
For the pool state storage, I'd implement Multi-Version Concurrency Control:
rustCopystruct MVCCPoolState {
versions: VecDeque<(u64, Arc<PoolState>)>, // (version, state)
current_version: u64,
}
impl MVCCPoolState {
fn read_at_version(&self, version: u64) -> Arc<PoolState> {
// Find the closest version less than or equal to requested version
let idx = self.versions.binary_search_by_key(&version, |(v, _)| *v)
.unwrap_or_else(|i| if i > 0 { i - 1 } else { 0 });
self.versions[idx].1.clone()
}
fn update(&mut self, new_state: PoolState) {
self.current_version += 1;
let new_version = Arc::new(new_state);
self.versions.push_back((self.current_version, new_version));
// Prune old versions beyond retention policy
while self.versions.len() > MAX_VERSIONS {
self.versions.pop_front();
}
}
}
Database Interaction Optimization
For database interactions, I'd use connection pooling and batch operations:
rustCopystruct DatabaseWriter {
pool: Pool<PostgresConnectionManager<NoTls>>,
batch_size: usize,
pending_writes: Vec<PoolUpdate>,
}
impl DatabaseWriter {
async fn write(&mut self, update: PoolUpdate) {
self.pending_writes.push(update);
if self.pending_writes.len() >= self.batch_size {
self.flush().await;
}
}
async fn flush(&mut self) {
let conn = self.pool.get().await.unwrap();
// Execute batch upsert
"INSERT INTO pool_states (pool_id, timestamp, liquidity, token_a, token_b)
let stmt = conn.prepare(
VALUES ($1, $2, $3, $4, $5)
ON CONFLICT (pool_id, timestamp) DO UPDATE
SET liquidity = EXCLUDED.liquidity,
token_a = EXCLUDED.token_a, token_b = EXCLUDED.token_b"
).await.unwrap();
// Create a transaction for the batch
let tx = conn.transaction().await.unwrap();
for update in &self.pending_writes {
tx.execute(&stmt, &[
&update.pool_id,
&update.timestamp,
&update.liquidity,
&update.token_a,
&update.token_b,
]).await.unwrap();
}
tx.commit().await.unwrap();
self.pending_writes.clear();
}
}
Monitoring and Adaptive Throttling
To ensure system stability, I'd implement adaptive throttling:
rustCopystruct AdaptiveThrottler {
current_tps: AtomicU64,
max_tps: AtomicU64,
system_load: Arc<Mutex<SystemLoad>>,
}
impl AdaptiveThrottler {
async fn should_process(&self, event: &BlockchainEvent) -> bool {
// Get current throughput and system metrics
let current = self.current_tps.load(Ordering::Relaxed);
let max = self.max_tps.load(Ordering::Relaxed);
let load = self.system_load.lock().await;
// If system is overloaded, dynamically adjust max TPS
if load.cpu_usage > 85.0 || load.memory_usage > 90.0 {
let new_max = (max as f64 * 0.9) as u64;
self.max_tps.store(new_max, Ordering::Relaxed);
// Apply priority-based throttling
if !is_high_priority(event) && current > new_max {
return false; // Throttle low-priority event
}
} else if load.cpu_usage < 60.0 && load.memory_usage < 70.0 {
// System has capacity, gradually increase max TPS
let new_max = (max as f64 * 1.05) as u64;
self.max_tps.store(new_max, Ordering::Relaxed);
}
true // Process the event
}
}
This comprehensive approach to concurrency addresses the challenges of high-throughput blockchain event processing while maintaining system stability and data integrity—essential qualities for a production system at Orca's scale.
Question
Answer this question "How would you implement a price impact calculation for an AMM swap?"
To implement a price impact calculation for an AMM swap, I'll create a comprehensive solution that accounts for both constant product AMMs and concentrated liquidity positions like those in Orca's Whirlpools.
Core Price Impact Implementation
For a standard constant product AMM (x * y = k), the price impact calculation involves comparing the execution price with the current spot price:
pub fn calculate_price_impact(
amount_in: u64,
token_in_reserve: u64,
token_out_reserve: u64,
slippage_tolerance: f64,
) -> Result<PriceImpactInfo, SwapError> {
// Validate inputs
if token_in_reserve == 0 || token_out_reserve == 0 {
return Err(SwapError::InsufficientLiquidity);
}
// Calculate the spot price before the swap
let spot_price = token_out_reserve as f64 / token_in_reserve as f64;
// Calculate the amount out using constant product formula (x * y = k)
let new_token_in_reserve = token_in_reserve.checked_add(amount_in)
.ok_or(SwapError::Overflow)?;
let new_token_out_reserve = (token_in_reserve as u128)
.checked_mul(token_out_reserve as u128)
.ok_or(SwapError::Overflow)?
.checked_div(new_token_in_reserve as u128)
.ok_or(SwapError::DivisionByZero)? as u64;
let amount_out = token_out_reserve.checked_sub(new_token_out_reserve)
.ok_or(SwapError::InsufficientOutputAmount)?;
// Calculate the execution price
let execution_price = amount_out as f64 / amount_in as f64;
// Calculate price impact as percentage difference between spot and execution price
let price_impact = ((spot_price - execution_price) / spot_price) * 100.0;
// Check if price impact exceeds slippage tolerance
let exceeds_slippage = price_impact > slippage_tolerance;
Ok(PriceImpactInfo {
spot_price,
execution_price,
price_impact,
amount_out,
exceeds_slippage,
})
}
Advanced CLAMM Price Impact Calculation
For Orca's Concentrated Liquidity AMMs (CLAMMs), the calculation is more complex as it must account for non-uniform liquidity distribution:
rustCopypub fn calculate_clamm_price_impact(
amount_in: u64,
token_in: &Token,
token_out: &Token,
pool: &WhirlpoolState,
tick_arrays: &[TickArray],
) -> Result<PriceImpactInfo, SwapError> {
// Get current sqrt price from the pool
let current_sqrt_price = pool.sqrt_price;
let current_tick = pool.tick_current_index;
// Determine swap direction
let a_to_b = token_in.mint == pool.token_mint_a;
// Get current spot price
let spot_price = calculate_price_from_sqrt_price(current_sqrt_price, a_to_b);
// Simulate the swap to find the ending price
let simulation = simulate_swap(
amount_in,
a_to_b,
current_sqrt_price,
current_tick,
pool.fee_rate,
tick_arrays,
)?;
// Calculate the execution price
let execution_price = simulation.amount_out as f64 / amount_in as f64;
// Calculate price impact
let price_impact = if a_to_b {
((spot_price - execution_price) / spot_price) * 100.0
} else {
((execution_price - spot_price) / spot_price) * 100.0
};
Ok(PriceImpactInfo {
spot_price,
execution_price,
price_impact,
amount_out: simulation.amount_out,
exceeds_slippage: price_impact > pool.slippage_tolerance,
})
}
fn simulate_swap(
amount_in: u64,
a_to_b: bool,
sqrt_price_current: u128,
tick_current: i32,
fee_rate: u16,
tick_arrays: &[TickArray],
) -> Result<SwapSimulation, SwapError> {
let fee_amount = calculate_fee(amount_in, fee_rate);
let amount_in_after_fee = amount_in.checked_sub(fee_amount)
.ok_or(SwapError::FeeCalculationFailure)?;
let mut remaining_amount = amount_in_after_fee;
let mut sqrt_price = sqrt_price_current;
let mut current_tick = tick_current;
let mut amount_out = 0;
// Process swap across tick boundaries, accounting for concentrated liquidity
while remaining_amount > 0 {
// Find the next initialized tick in the swap direction
let next_tick = find_next_initialized_tick(current_tick, a_to_b, tick_arrays)?;
// Calculate the sqrt price at this tick
let sqrt_price_target = calculate_sqrt_price_at_tick(next_tick);
// Calculate how much we can swap within the current price range
let (delta_in, delta_out, next_sqrt_price) = compute_swap_step(
sqrt_price,
sqrt_price_target,
pool.liquidity,
remaining_amount,
a_to_b,
)?;
// Update amounts
remaining_amount = remaining_amount.checked_sub(delta_in)
.ok_or(SwapError::CalculationFailure)?;
amount_out = amount_out.checked_add(delta_out)
.ok_or(SwapError::Overflow)?;
// Update price and tick
sqrt_price = next_sqrt_price;
// Cross the tick if we've reached it
if sqrt_price == sqrt_price_target {
current_tick = next_tick;
// Update liquidity when crossing a tick
let tick_data = get_tick_data(current_tick, tick_arrays)?;
pool.liquidity = update_liquidity_after_crossing(
pool.liquidity,
tick_data.net_liquidity_delta,
a_to_b,
);
} else {
// We didn't reach the next tick, so we're done
break;
}
}
Ok(SwapSimulation {
amount_out,
end_sqrt_price: sqrt_price,
end_tick: current_tick,
})
}
Optimization and Performance Considerations
For a high-performance system, I'd implement these optimizations:
// Precompute common price impacts for frequent swap sizes
struct PriceImpactCache {
cache: LruCache<SwapKey, PriceImpactInfo>,
}
impl PriceImpactCache {
fn get_or_compute(&mut self, key: SwapKey, pool: &PoolState) -> PriceImpactInfo {
if let Some(cached) = self.cache.get(&key) {
// Check if pool state has changed significantly
if !has_significant_state_change(&cached.pool_snapshot, pool) {
return cached.price_impact.clone();
}
}
// Compute new price impact
let price_impact = if pool.is_clamm {
calculate_clamm_price_impact(
key.amount_in,
&key.token_in,
&key.token_out,
&pool.whirlpool,
&pool.tick_arrays,
).unwrap_or_default()
} else {
calculate_price_impact(
key.amount_in,
pool.token_a_reserve,
pool.token_b_reserve,
pool.slippage_tolerance,
).unwrap_or_default()
};
// Cache the result
self.cache.put(key, CachedPriceImpact {
price_impact: price_impact.clone(),
pool_snapshot: take_pool_snapshot(pool),
timestamp: Instant::now(),
});
price_impact }
}
User-Facing Price Impact Display
For a complete solution, I'd provide user-friendly representations:
pub fn format_price_impact_for_display(impact: &PriceImpactInfo) -> String {
match impact.price_impact {
x if x < 0.1 => "Negligible impact (<0.1%)".to_string(),
x if x < 0.5 => format!("Low impact ({:.2}%)", x),
x if x < 1.0 => format!("Moderate impact ({:.2}%)", x),
x if x < 3.0 => format!("High impact ({:.2}%)", x),
x => format!("Very high impact ({:.2}%)! Caution advised.", x),
}
}
pub fn get_price_impact_color(impact: &PriceImpactInfo) -> &'static str {
match impact.price_impact {
x if x < 0.1 => "#00C853", // Green
x if x < 0.5 => "#AEEA00", // Light green
x if x < 1.0 => "#FFD600", // Yellow
x if x < 3.0 => "#FF9100", // Orange
x => "#FF3D00", // Red
}
}
This implementation provides:
- Accurate price impact calculations for both traditional AMMs and concentrated liquidity positions
- Performance optimizations through caching for frequently used swap sizes
- User-friendly visualization to help traders understand the implications
- Proper handling of edge cases and error conditions
The code is designed to be integrated into Orca's backend services to provide real-time price impact data for their frontend application or API consumers.
D
Answer this question "Describe your approach to error handling in Rust, especially when dealing with blockchain transactions that might fail.".
When dealing with blockchain transactions in Rust, error handling requires a thoughtful approach that balances robustness with clarity. Here's how I would implement error handling for blockchain transactions:
Comprehensive Error Type Hierarchy
I'd start by creating a domain-specific error type hierarchy:
#[derive(Debug, thiserror::Error)]
pub enum TransactionError {
#[error("RPC connection failed: {0}")]
ConnectionError(#[from] reqwest::Error),
#[error("Transaction simulation failed: {0}")]
SimulationFailure(String),
#[error("Transaction rejected: {0}")]
RejectedByNetwork(String),
#[error("Insufficient balance: required {required}, available {available}")]
InsufficientBalance { required: u64, available: u64 },
#[error("Instruction error at index {index}: {reason}")]
InstructionError { index: usize, reason: String },
#[error("Transaction timed out after {elapsed:?}")]
Timeout { elapsed: std::time::Duration },
#[error("Account not found: {0}")]
AccountNotFound(String),
#[error("Account data deserialization failed: {0}")]
DeserializationError(#[from] bincode::Error),
#[error("Slippage tolerance exceeded: expected {expected}, actual {actual}")]
SlippageExceeded { expected: f64, actual: f64 },
#[error("Internal error: {0}")]
Internal(String),
}
Context-Rich Result Propagation
I'd use context propagation throughout the codebase:
use anyhow::{Context, Result};
connection
pub async fn execute_swap(
connection: &RpcConnection,
pool_address: &Pubkey,
amount_in: u64,
min_amount_out: u64,
) -> Result<Signature> {
// Load accounts with context
let user_token_account = .get_token_account(&user_wallet.pubkey())
.await
.with_context(|| format!("Failed to load token account for user {}", user_wallet.pubkey()))?;
// Check balances with detailed errors
if user_token_account.amount < amount_in {
return Err(TransactionError::InsufficientBalance {
required: amount_in,
available: user_token_account.amount,
}.into());
}
// Simulate transaction before submitting
let transaction = build_swap_transaction(pool_address, amount_in, min_amount_out)
.with_context(|| "Failed to build swap transaction")?;
let simulation =
connection .simulate_transaction(&transaction)
.await
.with_context(|| "Failed to simulate transaction")?;
if let Some(err) = simulation.err {
// Parse and return specific error
return Err(parse_simulation_error(err)
.with_context(|| "Transaction simulation failed")?);
}
// Submit transaction with timeout
let signature = tokio::time::timeout(
Duration::from_secs(30),
connection.send_transaction(&transaction)
)
.await
.map_err(|_| TransactionError::Timeout { elapsed: Duration::from_secs(30)
})?
.with_context(|| "Failed to send transaction")?;
// Confirm transaction with custom retry logic
confirm_transaction_with_retry(connection, &signature, 5).await
}
Retry Logic with Exponential Backoff
For transient errors, I'd implement strategic retries:
rustCopyasync fn confirm_transaction_with_retry(
connection: &RpcConnection,
signature: &Signature,
max_retries: usize,
) -> Result<()> {
let mut backoff = Duration::from_millis(500);
for attempt in 0..max_retries {
err
match connection.confirm_transaction(signature).await {
Ok(confirmed) => {
if confirmed {
// Transaction confirmed
return Ok(());
}
}
Err(err) => {
if is_transient_error(&err) && attempt < max_retries - 1 {
// Log the transient error but continue retrying
tracing::warn!(
"Transient error confirming transaction {}, retry {}/{}: {}",
signature, attempt + 1, max_retries, );
// Exponential backoff with jitter
let jitter = rand::thread_rng().gen_range(0..100);
tokio::time::sleep(backoff + Duration::from_millis(jitter)).await;
backoff *= 2; // Exponential backoff
continue;
}
// Non-transient error or max retries reached
return Err(err).with_context(|| format!(
"Failed to confirm transaction {} after {} attempts", signature, attempt + 1
));
}
}
// Transaction not yet confirmed, wait and retry
tokio::time::sleep(backoff).await;
}
Err(TransactionError::Timeout {
elapsed: backoff * max_retries as u32
}.into())
}
fn is_transient_error(err: &reqwest::Error) -> bool {
err.is_timeout() || err.is_connect() || (err.status().map_or(false, |s| s.as_u16() >= 500))
}
Result Combinators for Cleaner Code
I'd use result combinators for concise error handling:
pub async fn process_pool_events(
events: Vec<PoolEvent>,
db: &DatabasePool,
) -> Result<ProcessingSummary> {
let results = futures::future::join_all(
events.into_iter().map(|event| process_single_event(event, db))
).await;
// Count successes and collect errors
let (processed, errors): (Vec<_>, Vec<_>) = results.into_iter()
.partition(Result::is_ok);
let processed_count = processed.len();
let error_count = errors.len();
// Log errors in detail
for error in &errors {
if let Err(e) = error {
tracing::error!("Event processing error: {:?}", e);
}
}
// If all failed, return error; otherwise return summary
if processed_count == 0 && error_count > 0 {
Err(anyhow::anyhow!("All {} events failed processing", error_count))
} else {
Ok(ProcessingSummary {
processed_count,
error_count,
// Map first few errors for reporting
sample_errors: errors.into_iter()
.take(5)
.map(|e| format!("{:?}", e))
.collect(),
})
}
}
Transaction-Specific Error Recovery
For blockchain-specific errors, I'd implement specialized recovery:
async fn execute_transaction_with_recovery(
connection: &RpcConnection,
transaction: Transaction,
) -> Result<Signature> {
match connection.send_transaction(&transaction).await {
Ok(signature) => Ok(signature),
Err(err) => match err {
TransactionError::RejectedByNetwork(reason) if reason.contains("blockhash not found") => {
// Blockhash expired, refresh and retry once
tracing::info!("Refreshing blockhash and retrying transaction");
let new_blockhash = connection.get_latest_blockhash().await?;
let updated_tx = transaction.with_blockhash(new_blockhash);
connection.send_transaction(&updated_tx).await
.with_context(|| "Failed to send transaction with refreshed blockhash")
},
TransactionError::InstructionError { index, reason }
if reason.contains("insufficient funds") => {
// Check if this is a fee-related issue
if index == 0 && transaction.instructions[0].program_id == system_program::ID {
// Try adding a fee payer
tracing::info!("Adding fee payer to transaction");
let fee_payer = get_fee_payer()?;
let updated_tx = add_fee_payer(transaction, &fee_payer);
connection.send_transaction(&updated_tx).await
.with_context(|| "Failed to send transaction with fee payer")
} else {
Err(err.into())
}
},
// For other errors, propagate them
_ => Err(err.into()),
}
}
}
Graceful Degradation
For a production system, I'd implement graceful degradation:
impl LiquidityPoolService {
pub async fn get_pool_data(&self, pool_id: &Pubkey) -> Result<PoolData> {
// Try primary data source first (on-chain)
match self.load_pool_from_chain(pool_id).await {
Ok(data) => {
// Cache successful result
self.cache.insert(pool_id, data.clone());
Ok(data)
}
Err(err) => {
tracing::warn!("Failed to load pool from chain: {:?}", err);
// Try backup data sources in order
if let Some(cached) = self.cache.get(pool_id) {
tracing::info!("Using cached pool data (may be slightly stale)");
return Ok(cached.clone());
}
// Try database backup
match self.db.get_latest_pool_state(pool_id).await {
Ok(db_state) => {
tracing::info!("Using database backup for pool data");
Ok(db_state)
}
Err(db_err) => {
db_err
// All sources failed, return original error with context
Err(err).with_context(|| format!(
"All data sources failed for pool {}: DB error: {}",
pool_id, ))
}
}
}
}
}
}
Telemetry and Monitoring
I'd ensure all errors are properly instrumented:
// Before submitting a transaction
let tx_id = uuid::Uuid::new_v4();
// Start a span for this transaction
let span = tracing::info_span!(
"process_swap",
tx_id = %tx_id,
pool_id = %pool_id,
user = %user.pubkey(),
amount_in = amount_in,
);
// Execute with the span active
let result = async {
let start = std::time::Instant::now();
let result = execute_swap(connection, pool_id, amount_in, min_amount_out).await;
// Record metrics
metrics::histogram!("swap.duration", start.elapsed());
match &result {
Ok(_) => {
metrics::counter!("swap.success", 1);
}
Err(e) => {
metrics::counter!("swap.error", 1, "error_type" => error_type(e));
}
}
result}.instrument(span).await;
This comprehensive approach to error handling ensures:
- Clarity: Errors are specific and informative
- Recovery: Transient failures can be automatically retried
- Graceful Degradation: The system provides the best possible response even during failures
- Observability: Errors are logged and metrics captured for monitoring
- User Experience: Users receive actionable information about failures
In blockchain systems, where transactions can fail for various reasons beyond our control, robust error handling is essential for maintaining reliability and user trust.
Question
Answer this question "How would you design a database schema to track historical liquidity and volume for thousands of trading pairs?"
Database Schema for Historical Liquidity and Volume Tracking
To design a database schema that effectively tracks historical liquidity and volume for thousands of trading pairs, I'd implement a time-series optimized structure that balances query performance with storage efficiency.
Core Tables
1. Trading Pairs Table
CREATE TABLE trading_pairs (
pair_id SERIAL PRIMARY KEY,
base_token_mint VARCHAR(44) NOT NULL,
quote_token_mint VARCHAR(44) NOT NULL,
pair_address VARCHAR(44) UNIQUE NOT NULL,
pair_name VARCHAR(100) NOT NULL,
is_stable BOOLEAN NOT NULL DEFAULT FALSE,
created_at TIMESTAMPTZ NOT NULL DEFAULT NOW(),
last_active_at TIMESTAMPTZ NOT NULL DEFAULT NOW(),
UNIQUE (base_token_mint, quote_token_mint)
);
CREATE INDEX idx_trading_pairs_tokens ON trading_pairs(base_token_mint, quote_token_mint);
CREATE INDEX idx_trading_pairs_address ON trading_pairs(pair_address);
2. Liquidity Snapshots Table
CREATE TABLE liquidity_snapshots (
snapshot_id BIGSERIAL PRIMARY KEY,
pair_id INTEGER NOT NULL REFERENCES trading_pairs(pair_id),
timestamp TIMESTAMPTZ NOT NULL,
base_reserve NUMERIC(36, 18) NOT NULL,
quote_reserve NUMERIC(36, 18) NOT NULL,
liquidity_usd NUMERIC(36, 2),
price NUMERIC(36, 18) NOT NULL,
tick_lower INTEGER, -- For concentrated liquidity pools
tick_upper INTEGER, -- For concentrated liquidity pools
fee_tier SMALLINT
);
-- Convert to TimescaleDB hypertable
SELECT create_hypertable('liquidity_snapshots', 'timestamp', chunk_time_interval => INTERVAL '1 day');
-- Indexes for efficient queries
CREATE INDEX idx_liquidity_pair_time ON liquidity_snapshots(pair_id, timestamp DESC);
CREATE INDEX idx_liquidity_time ON liquidity_snapshots(timestamp DESC);
3. Volume Data Table
sqlCopyCREATE TABLE volume_data (
volume_id BIGSERIAL PRIMARY KEY,
pair_id INTEGER NOT NULL REFERENCES trading_pairs(pair_id),
timestamp TIMESTAMPTZ NOT NULL,
base_volume NUMERIC(36, 18) NOT NULL,
quote_volume NUMERIC(36, 18) NOT NULL,
volume_usd NUMERIC(36, 2),
transaction_count INTEGER NOT NULL,
unique_users INTEGER,
avg_trade_size_usd NUMERIC(36, 2),
largest_trade_usd NUMERIC(36, 2)
);
-- Convert to TimescaleDB hypertable
SELECT create_hypertable('volume_data', 'timestamp', chunk_time_interval => INTERVAL '1 hour');
-- Indexes for common queries
CREATE INDEX idx_volume_pair_time ON volume_data(pair_id, timestamp DESC);
CREATE INDEX idx_volume_time ON volume_data(timestamp DESC);
4. Price Candles Table
sqlCopyCREATE TABLE price_candles (
candle_id BIGSERIAL PRIMARY KEY,
pair_id INTEGER NOT NULL REFERENCES trading_pairs(pair_id),
timestamp TIMESTAMPTZ NOT NULL,
interval_length VARCHAR(10) NOT NULL, -- '1m', '5m', '1h', '1d', etc.
open_price NUMERIC(36, 18) NOT NULL,
high_price NUMERIC(36, 18) NOT NULL,
low_price NUMERIC(36, 18) NOT NULL,
close_price NUMERIC(36, 18) NOT NULL,
volume NUMERIC(36, 18) NOT NULL
);
-- Convert to TimescaleDB hypertable
SELECT create_hypertable('price_candles', 'timestamp', chunk_time_interval => INTERVAL '1 day');
-- Create unique constraint for interval data
CREATE UNIQUE INDEX idx_candles_unique ON price_candles(pair_id, timestamp, interval_length);
Aggregation and Rollup Tables
1. Daily Pair Statistics
sqlCopyCREATE TABLE daily_pair_stats (
daily_pair_stats_mv
stats_id BIGSERIAL PRIMARY KEY,
pair_id INTEGER NOT NULL REFERENCES trading_pairs(pair_id),
day DATE NOT NULL,
open_price NUMERIC(36, 18) NOT NULL,
close_price NUMERIC(36, 18) NOT NULL,
high_price NUMERIC(36, 18) NOT NULL,
low_price NUMERIC(36, 18) NOT NULL,
volume_usd NUMERIC(36, 2) NOT NULL,
volume_base NUMERIC(36, 18) NOT NULL,
volume_quote NUMERIC(36, 18) NOT NULL,
liquidity_usd NUMERIC(36, 2) NOT NULL,
trade_count INTEGER NOT NULL,
unique_traders INTEGER NOT NULL,
UNIQUE (pair_id, day)
);
-- Create continuosly updated materialized view
CREATE MATERIALIZED VIEWWITH (timescaledb.continuous) AS
SELECT pair_id,
unique_traders
time_bucket('1 day', timestamp) AS day,
first(open_price, timestamp) AS open_price,
last(close_price, timestamp) AS close_price,
max(high_price) AS high_price,
min(low_price) AS low_price,
sum(volume_usd) AS volume_usd,
sum(base_volume) AS volume_base,
sum(quote_volume) AS volume_quote,
avg(liquidity_usd) AS liquidity_usd,
sum(transaction_count) AS trade_count,
sum(unique_users) ASFROM
price_candles pcJOIN
volume_data vd USING (pair_id, timestamp)
JOIN liquidity_snapshots ls USING (pair_id, timestamp)
GROUP BY pair_id, day
WITH NO DATA;
-- Refresh policy
SELECT add_continuous_aggregate_policy('daily_pair_stats_mv',
start_offset => INTERVAL '3 days',
end_offset => INTERVAL '1 hour',
schedule_interval => INTERVAL '1 hour');
Additional Supporting Tables
1. Token Information
sqlCopyCREATE TABLE tokens (
token_mint VARCHAR(44) PRIMARY KEY,
token_name VARCHAR(100) NOT NULL,
token_symbol VARCHAR(20) NOT NULL,
decimals SMALLINT NOT NULL,
coingecko_id VARCHAR(100),
logo_url TEXT,
is_stable BOOLEAN DEFAULT FALSE,
created_at TIMESTAMPTZ NOT NULL DEFAULT NOW()
);
2. USD Price History
sqlCopyCREATE TABLE token_usd_prices (
price_id BIGSERIAL PRIMARY KEY,
token_mint VARCHAR(44) NOT NULL REFERENCES tokens(token_mint),
timestamp TIMESTAMPTZ NOT NULL,
price_usd NUMERIC(36, 18) NOT NULL
);
-- Convert to TimescaleDB hypertable
SELECT create_hypertable('token_usd_prices', 'timestamp', chunk_time_interval => INTERVAL '1 day');
-- Create index
CREATE INDEX idx_token_price_time ON token_usd_prices(token_mint, timestamp DESC);
Compression and Retention Policies
sqlCopy-- Compress chunks older than 7 days
ALTER TABLE liquidity_snapshots SET (
timescaledb.compress,
timescaledb.compress_segmentby = 'pair_id'
);
SELECT add_compression_policy('liquidity_snapshots', INTERVAL '7 days');
-- Set retention policy for high resolution data
SELECT add_retention_policy('liquidity_snapshots', INTERVAL '90 days');
SELECT add_retention_policy('volume_data', INTERVAL '90 days');
-- Keep aggregated data much longer
SELECT add_retention_policy('daily_pair_stats', INTERVAL '10 years');
Design Rationale
- TimescaleDB Hypertables: Using TimescaleDB optimizes time-series data storage and retrieval, with automatic partitioning by time chunks.
- Multiple Resolution Storage:
- High-resolution data (minute/hour level) for recent history
- Aggregated data (daily) for long-term trends
- Compression for older data
- Separation of Concerns:
- Volume data separate from liquidity data
- Price candles independent of volume for specialized queries
- Continuous Aggregation:
- Materialized views that update automatically
- Efficient querying of aggregated historical data
- Optimization for Common Queries:
- Pool comparison queries
- Time-range analysis
- Protocol-wide statistics
Scaling Considerations
For handling thousands of trading pairs with years of historical data:
- Partitioning Strategy:
- Time-based partitioning with TimescaleDB
- Additional partitioning by pair activity level
- Query Optimization:
- Pre-calculated aggregates for common time windows
- Caching layer for frequent queries
- Hardware Configuration:
- Main database with high-performance SSD storage
- Read replicas for analytics queries
- Separate instances for real-time vs. historical data
This schema design balances the need for granular historical data with query performance and storage efficiency, making it suitable for Orca's requirements to analyze thousands of trading pairs across the Solana ecosystem.
Question
Answer this question "How would you design a high-performance event processor for Solana transactions?"
Designing a High-Performance Solana Transaction Event Processor
To design a high-performance event processor for Solana transactions, I'd create a multi-layered architecture optimized for throughput, reliability, and low latency.
System Architecture Overview
Copy[Solana Validators] → [Data Ingestion Layer] → [Processing Pipeline] → [Storage Layer] → [API/Service Layer]
1. Data Ingestion Layer
Geyser Plugin Integration
rustCopypub struct CustomGeyserPlugin {
event_sender: mpsc::Sender<SolanaEvent>,
config: PluginConfig,
metrics: Arc<Metrics>,
}
impl GeyserPlugin for CustomGeyserPlugin {
fn on_account_update(&self, account: ReplicaAccountInfoVersions, slot: u64, is_startup: bool) {
// Filter accounts of interest (e.g., only specific programs or account types)
if !self.should_process_account(&account) {
return;
}
let account_data = self.extract_account_data(account);
// Send to processing pipeline with backpressure awareness
if let Err(e) = self.event_sender.try_send(SolanaEvent::AccountUpdate { slot, account_data,
is_startup }) {
self.metrics.dropped_events.inc();
if e.is_full() {
// Handle backpressure - increment separate metric
self.metrics.backpressure_events.inc();
}
}
}
fn on_transaction(&self, transaction: ReplicaTransactionInfoVersions, slot: u64) {
// Filter for relevant transactions
if !self.should_process_transaction(&transaction) {
return;
}
let tx_data = self.extract_transaction_data(transaction);
// Send to processing pipeline
if let Err(_) = self.event_sender.try_send(SolanaEvent::Transaction { slot,
tx_data }) {
self.metrics.dropped_transactions.inc();
}
}
}
Yellowstone gRPC Client
rustCopypub async fn run_yellowstone_client(
config: YellowstoneConfig,
event_sender: mpsc::Sender<SolanaEvent>,
) -> Result<(), ClientError> {
let connection_config = yellowstone_grpc::ClientConfig {
url: config.grpc_url.clone(),
timeout: Some(Duration::from_secs(60)),
retry_delay: Duration::from_secs(1),
max_retries: 10,
};
let mut client = yellowstone_grpc::GeyserGrpcClient::connect(connection_config).await?;
// Subscribe to specific accounts and transaction filters
let subscribe_request = yellowstone_grpc::SubscribeRequest {
accounts: Some(yellowstone_grpc::SubscribeRequestFilterAccounts {
account_includes: config.tracked_accounts.clone(),
..Default::default()
}),
transactions: Some(yellowstone_grpc::SubscribeRequestFilterTransactions {
vote: false,
failed: false,
signatures: vec![],
accounts: config.program_ids.clone(),
}),
..Default::default()
};
let mut subscription = client.subscribe(subscribe_request).await?;
while let Some(message) = subscription.message().await? {
match message {
yellowstone_grpc::SubscribeUpdate::Account(account) => {
let event = SolanaEvent::AccountUpdate {
slot: account.slot,
account_data: AccountData {
pubkey: account.pubkey,
data: account.data,
owner: account.owner,
lamports: account.lamports,
},
is_startup: false,
};
if let Err(_) = event_sender.send(event).await {
return Err(ClientError::SendError);
}
},
yellowstone_grpc::SubscribeUpdate::Transaction(transaction) => {
// Process transaction data
if let Err(_) = process_and_send_transaction(transaction, &event_sender).await {
return Err(ClientError::SendError);
}
},
_ => { /* Ignore other update types */ }
}
}
Ok(())
}
2. Processing Pipeline
Event Dispatcher and Work Stealing
rustCopypub struct EventDispatcher {
worker_pool: Vec<mpsc::Sender<WorkItem>>,
metrics: Arc<Metrics>,
pool_size: usize,
}
impl EventDispatcher {
pub fn new(pool_size: usize) -> (Self, Vec<mpsc::Receiver<WorkItem>>) {
let mut senders = Vec::with_capacity(pool_size);
let mut receivers = Vec::with_capacity(pool_size);
for _ in 0..pool_size {
let (tx, rx) = mpsc::channel(10_000);
senders.push(tx);
receivers.push(rx);
}
let dispatcher = Self {
worker_pool: senders,
metrics: Arc::new(Metrics::default()),
pool_size,
};
(dispatcher, receivers)
}
pub async fn dispatch(&self, event: SolanaEvent) -> Result<(), DispatchError> {
// Determine which worker should process this event
let worker_idx = match &event {
SolanaEvent::AccountUpdate { account_data, .. } => {
// Consistent hashing to ensure same account always goes to same worker
let hash = seahash::hash(account_data.pubkey.as_ref());
(hash % self.pool_size as u64) as usize
}
SolanaEvent::Transaction { tx_data, .. } => {
// Distribute transactions evenly
let sig_bytes = tx_data.signature.as_ref();
let hash = seahash::hash(sig_bytes);
(hash % self.pool_size as u64) as usize
}
};
// Send to selected worker
let work_item = WorkItem::new(event);
if let Err(_) = self.worker_pool[worker_idx].send(work_item).await {
self.metrics.dispatch_failures.inc();
return Err(DispatchError::WorkerUnavailable);
}
Ok(())
}
}
// Work stealing implementation for load balancing
pub async fn run_work_stealing(
worker_id: usize,
my_receiver: &mut mpsc::Receiver<WorkItem>,
other_workers: Vec<WorkStealingHandle>,
processor: EventProcessor,
) {
let mut backoff = ExponentialBackoff::default();
loop {
// First try our own queue
match my_receiver.try_recv() {
Ok(work) => {
backoff.reset();
processor.process(work).await;
continue;
}
Err(TryRecvError::Empty) => {
// Our queue is empty, try to steal work
}
Err(TryRecvError::Disconnected) => {
// Channel closed, exit
break;
}
}
// Try to steal work from other workers
let mut stole_work = false;
for handle in &other_workers {
if let Some(work) = handle.try_steal().await {
processor.process(work).await;
stole_work = true;
break;
}
}
if !stole_work {
// No work found, back off before trying again
let backoff_duration = backoff.next_backoff().unwrap_or(Duration::from_millis(100));
tokio::time::sleep(backoff_duration).await;
}
}
}
Specialized Event Processor
rustCopypub struct EventProcessor {
pool_state_cache: LruCache<Pubkey, Arc<RwLock<PoolState>>>,
tx_parser: TransactionParser,
db_writer: DatabaseWriter,
metrics: Arc<Metrics>,
}
impl EventProcessor {
pub async fn process(&mut self, work_item: WorkItem) {
let start = Instant::now();
match work_item.event {
SolanaEvent::AccountUpdate { account_data, slot, .. } => {
if let Some(pool_state) = self.tx_parser.parse_pool_account(&account_data) {
// Update in-memory cache
self.update_pool_state(account_data.pubkey, pool_state, slot).await;
self.metrics.account_updates_processed.inc();
}
}
SolanaEvent::Transaction { tx_data, slot } => {
// Process the transaction
match self.tx_parser.parse_transaction(&tx_data) {
Ok(tx_events) => {
for event in tx_events {
match event {
ParsedEvent::Swap { pool_id, amount_in, amount_out, .. } => {
self.record_swap(pool_id, amount_in, amount_out, slot).await;
self.metrics.swaps_processed.inc();
}
ParsedEvent::Deposit { pool_id, liquidity_added, .. } => {
self.record_deposit(pool_id, liquidity_added, slot).await;
self.metrics.deposits_processed.inc();
}
ParsedEvent::Withdraw { pool_id, liquidity_removed, .. } => {
self.record_withdrawal(pool_id, liquidity_removed, slot).await;
self.metrics.withdrawals_processed.inc();
}
}
}
}
Err(e) => {
self.metrics.tx_parse_errors.inc();
tracing::warn!("Failed to parse transaction: {}", e);
}
}
}
}
let elapsed = start.elapsed();
self.metrics.processing_time.observe(elapsed.as_millis() as f64);
}
async fn update_pool_state(&mut self, pool_id: Pubkey, new_state: PoolState, slot: u64) {
pool_state_cache
// Get or create pool state entry
let state_entry = self. .entry(pool_id)
.or_insert_with(|| Arc::new(RwLock::new(PoolState::default())));
// Update in-memory state
{
let mut state = state_entry.write().await;
// Only update if new data is from a newer slot
if slot >= state.last_update_slot {
*state = new_state;
state.last_update_slot = slot;
// Write to database asynchronously
let state_clone = state.clone();
self.db_writer.write_pool_state(pool_id, state_clone, slot);
}
}
}
}
3. Optimized Storage Layer
Tiered Storage Strategy
rustCopypub struct DatabaseWriter {
in_memory_buffer: RwLock<HashMap<Pubkey, Vec<PoolStateUpdate>>>,
timescale_pool: deadpool_postgres::Pool,
buffer_size_limit: usize,
last_flush: AtomicU64,
flush_interval: Duration,
}
impl DatabaseWriter {
pub fn write_pool_state(&self, pool_id: Pubkey, state: PoolState, slot: u64) {
// Add to in-memory buffer first
let update = PoolStateUpdate {
pool_id,
state,
slot,
timestamp: SystemTime::now(),
};
{
let mut buffer = self.in_memory_buffer.write().unwrap();
buffer.entry(pool_id)
.or_insert_with(Vec::new)
.push(update);
}
// Check if we should flush to database
let buffer_size = {
let buffer = self.in_memory_buffer.read().unwrap();
buffer.values().map(|v| v.len()).sum::<usize>()
};
let last_flush = self.last_flush.load(Ordering::Relaxed);
let now = SystemTime::now()
.duration_since(UNIX_EPOCH)
.unwrap()
.as_secs();
if buffer_size > self.buffer_size_limit || now - last_flush > self.flush_interval.as_secs() {
// Spawn task to flush to database
let writer = self.clone();
tokio::spawn(async move {
if let Err(e) = writer.flush_to_database().await {
tracing::error!("Failed to flush to database: {}", e);
}
});
}
}
async fn flush_to_database(&self) -> Result<(), DbError> {
// Take the current buffer
let buffer = {
let mut buffer_guard = self.in_memory_buffer.write().unwrap();
std::mem::take(&mut *buffer_guard)
};
if buffer.is_empty() {
return Ok(());
}
// Group updates by pool for batch inserts
let mut pool_updates: HashMap<Pubkey, Vec<PoolStateUpdate>> = HashMap::new();
for (pool_id, updates) in buffer {
pool_updates.insert(pool_id, updates);
}
// Get connection from pool
let conn = self.timescale_pool.get().await?;
// Start transaction
let tx = conn.transaction().await?;
for (pool_id, updates) in pool_updates {
"INSERT INTO pool_states
// Batch insert pool state updates
let stmt = tx.prepare_cached(
(pool_id, slot, timestamp, liquidity, token_a_amount, token_b_amount,
sqrt_price, tick_current, fee_growth_global_a, fee_growth_global_b) VALUES ($1, $2, $3, $4, $5, $6, $7, $8, $9, $10)"
).await?;
for update in updates {
tx.execute(&stmt, &[
&pool_id.to_string(),
&(update.slot as i64),
&update.timestamp,
&update.state.liquidity.to_string(),
&update.state.token_a_amount.to_string(),
&update.state.token_b_amount.to_string(),
&update.state.sqrt_price.to_string(),
&(update.state.tick_current as i32),
&update.state.fee_growth_global_a.to_string(),
&update.state.fee_growth_global_b.to_string(),
]).await?;
}
}
// Commit transaction
tx.commit().await?;
// Update last flush timestamp
let now = SystemTime::now()
.duration_since(UNIX_EPOCH)
.unwrap()
.as_secs();
self.last_flush.store(now, Ordering::Relaxed);
Ok(())
}
}
4. Performance Optimizations
Prefetching and Caching
rustCopypub struct PrefetchingCache {
pool_states: LruCache<Pubkey, Arc<PoolState>>,
access_frequency: HashMap<Pubkey, AtomicU64>,
db_client: Arc<DatabaseClient>,
}
impl PrefetchingCache {
pub async fn get_pool_state(&mut self, pool_id: &Pubkey) -> Result<Arc<PoolState>, CacheError> {
// Try to get from cache first
if let Some(state) = self.pool_states.get(pool_id) {
// Update access count
self.record_access(pool_id);
return Ok(state.clone());
}
// Not in cache, fetch from database
let state = self.db_client.get_latest_pool_state(pool_id).await?;
let state_arc = Arc::new(state);
// Add to cache
self.pool_states.put(*pool_id, state_arc.clone());
// Start prefetching related pools
self.prefetch_related_pools(pool_id);
Ok(state_arc)
}
fn record_access(&mut self, pool_id: &Pubkey) {
access_frequency
let counter = self. .entry(*pool_id)
.or_insert_with(|| AtomicU64::new(0));
counter.fetch_add(1, Ordering::Relaxed);
}
fn prefetch_related_pools(&self, pool_id: &Pubkey) {
// Spawn background task to prefetch related pools
let db_client = self.db_client.clone();
let pool_id = *pool_id;
tokio::spawn(async move {
// Find related pools (e.g., with same token)
match db_client.find_related_pools(&pool_id).await {
Ok(related_pools) => {
for related_id in related_pools {
// Prefetch in background
if let Err(e) = db_client.get_latest_pool_state(&related_id).await {
tracing::debug!("Failed to prefetch related pool {}: {}", related_id, e);
}
}
}
Err(e) => {
tracing::debug!("Failed to find related pools for {}: {}", pool_id, e);
}
}
});
}
}
Zero-Copy Event Processing
rustCopypub struct ZeroCopyEventParser {
pool_id_to_layout: HashMap<Pubkey, AccountLayout>,
}
impl ZeroCopyEventParser {
pub fn parse_account_data(&self, data: &[u8], owner: &Pubkey) -> Result<ParsedAccount, ParseError> {
// Find appropriate layout based on owner program
let layout = match self.get_layout_for_program(owner) {
Some(layout) => layout,
None => return Err(ParseError::UnsupportedProgram(*owner)),
};
// Use zero-copy deserialization
match layout {
AccountLayout::Whirlpool => {
// Cast the data slice directly to the struct without copying
if data.len() < std::mem::size_of::<WhirlpoolState>() {
return Err(ParseError::InvalidDataSize);
}
let state = unsafe {
// SAFETY: We've verified the data size above
&*(data.as_ptr() as *const WhirlpoolState)
};
// Extract only the fields we need
Ok(ParsedAccount::Whirlpool(WhirlpoolData {
sqrt_price: state.sqrt_price,
liquidity: state.liquidity,
tick_current_index: state.tick_current_index,
token_mint_a: state.token_mint_a,
token_mint_b: state.token_mint_b,
fee_rate: state.fee_rate,
}))
}
AccountLayout::TokenAccount => {
// Direct cast for SPL Token accounts
if data.len() < std::mem::size_of::<TokenAccountState>() {
return Err(ParseError::InvalidDataSize);
}
let state = unsafe {
&*(data.as_ptr() as *const TokenAccountState)
};
Ok(ParsedAccount::TokenAccount(TokenAccountData {
mint: state.mint,
owner: state.owner,
amount: state.amount,
}))
}
// Other account layouts...
}
}
}
5. Reliability and Fault Tolerance
Circuit Breaker and Fallback Mechanisms
rustCopypub struct RpcConnectionManager {
primary_connections: Vec<RpcConnection>,
fallback_connections: Vec<RpcConnection>,
circuit_breakers: HashMap<RpcEndpoint, CircuitBreaker>,
}
impl RpcConnectionManager {
pub async fn get_connection(&self) -> Result<&RpcConnection, ConnectionError> {
// Try primary connections first
for conn in &self.primary_connections {
let endpoint = &conn.endpoint;
// Check if circuit breaker is open
if let Some(breaker) = self.circuit_breakers.get(endpoint) {
if breaker.is_open() {
// Skip this connection, circuit is open
continue;
}
}
// Test connection with ping
match timeout(Duration::from_millis(500), conn.ping()).await {
Ok(Ok(_)) => {
// Connection is healthy
return Ok(conn);
}
_ => {
// Mark connection as unhealthy
if let Some(breaker) = self.circuit_breakers.get(endpoint) {
breaker.record_failure();
}
}
}
}
// Try fallback connections if all primary failed
for conn in &self.fallback_connections {
if conn.is_available() {
return Ok(conn);
}
}
Err(ConnectionError::NoAvailableConnections)
}
}
pub struct CircuitBreaker {
failures: AtomicUsize,
last_failure: AtomicU64,
threshold: usize,
reset_timeout: Duration,
}
impl CircuitBreaker {
pub fn is_open(&self) -> bool {
let failures = self.failures.load(Ordering::Relaxed);
if failures < self.threshold {
return false;
}
// Check if we've waited long enough to try again
let last_failure = self.last_failure.load(Ordering::Relaxed);
let now = SystemTime::now()
.duration_since(UNIX_EPOCH)
.unwrap()
.as_secs();
now - last_failure < self.reset_timeout.as_secs()
}
pub fn record_failure(&self) {
self.failures.fetch_add(1, Ordering::Relaxed);
let now = SystemTime::now()
.duration_since(UNIX_EPOCH)
.unwrap()
.as_secs();
self.last_failure.store(now, Ordering::Relaxed);
}
pub fn record_success(&self) {
// Reset failure count on successful operation
self.failures.store(0, Ordering::Relaxed);
}
}
Monitoring and Observability
rustCopypub struct MetricsReporter {
metrics: Arc<Metrics>,
registry: prometheus::Registry,
report_interval: Duration,
}
impl MetricsReporter {
pub async fn run(&self) {
let mut interval = tokio::time::interval(self.report_interval);
loop {
interval.tick().await;
// Gather metrics
let events_processed = self.metrics.events_processed.get();
let processing_time_p95 = self.metrics.processing_time.quantile(0.95).unwrap_or(0.0);
let current_lag = self.calculate_processing_lag();
// Log key metrics
tracing::info!(
"Events processed: {}, P95 latency: {:.2}ms, Processing lag: {}ms",
events_processed,
processing_time_p95,
current_lag.as_millis()
);
// Send to monitoring system
if let Err(e) = self.export_metrics().await {
tracing::error!("Failed to export metrics: {}", e);
}
}
}
fn calculate_processing_lag(&self) -> Duration {
let latest_slot_seen = self.metrics.latest_slot_seen.get();
let latest_slot_processed = self.metrics.latest_slot_processed.get();
let slot_diff = latest_slot_seen.saturating_sub(latest_slot_processed);
// Convert slot difference to time (average 400ms per slot on Solana)
Duration::from_millis(slot_diff * 400)
}
}
Complete System Integration
rustCopypub async fn run_event_processor(config: Config) -> Result<(), ProcessorError> {
// Set up metrics
let metrics = Arc::new(Metrics::new(&config.metrics_prefix));
// Set up connection management
let connection_manager = RpcConnectionManager::new(config.rpc_endpoints).await?;
// Create channel for event processing
let (event_sender, event_receiver) = mpsc::channel(config.channel_capacity);
// Start data ingestion based on configuration
if config.use_geyser_plugin {
let plugin_config = GeyserPluginConfig::from_file(&config.plugin_config_path)?;
let plugin = CustomGeyserPlugin::new(event_sender.clone(), plugin_config, metrics.clone());
// Register plugin with validator (implementation specific)
// ...
} else {
// Use Yellowstone gRPC client
let yellowstone_config = YellowstoneConfig::new(
config.grpc_url.clone(),
config.tracked_accounts.clone(),
config.program_ids.clone(),
);
tokio::spawn(run_yellowstone_client(yellowstone_config, event_sender));
}
// Set up worker pool
let pool_size = config.worker_threads.unwrap_or_else(num_cpus::get);
let (dispatcher, receivers) = EventDispatcher::new(pool_size);
// Start event dispatcher
let dispatcher_metrics = metrics.clone();
tokio::spawn(async move {
let mut event_receiver = event_receiver;
while let Some(event) = event_receiver.recv().await {
if let Err(e) = dispatcher.dispatch(event).await {
tracing::error!("Failed to dispatch event: {}", e);
dispatcher_metrics.dispatch_errors.inc();
}
}
});
// Start worker threads
let mut worker_handles = Vec::with_capacity(pool_size);
for (worker_id, receiver) in receivers.into_iter().enumerate() {
let worker_metrics = metrics.clone();
let db_pool = create_database_pool(&config.database_url, config.db_pool_size).await?;
let processor = EventProcessor::new(
db_pool,
worker_metrics.clone(),
connection_manager.clone(),
);
let handle = tokio::spawn(async move {
tracing::info!("Starting worker {}", worker_id);
let mut receiver = receiver;
while let Some(work_item) = receiver.recv().await {
processor.process(work_item).await;
}
tracing::info!("Worker {} shutting down", worker_id);
});
worker_handles.push(handle);
}
// Start metrics reporter
let reporter = MetricsReporter::new(metrics.clone(), config.metrics_interval);
tokio::spawn(reporter.run());
// Wait for all workers to complete
for handle in worker_handles {
if let Err(e) = handle.await {
tracing::error!("Worker thread panicked: {}", e);
}
}
Ok(())
}
Key Design Aspects
- Horizontal Scalability:
- Sharded processing based on account/transaction hash
- Work stealing for load balancing
- Multi-tiered storage for different access patterns
- Minimal Copying and Zero-Copy Parsing:
- Direct deserialization from account data where possible
- Efficient event representation minimizing allocations
- Optimized State Management:
- In-memory caching of active pools
- Prefetching based on access patterns
- Batched database writes
- Fault Tolerance:
- Circuit breakers for RPC endpoints
- Message retries with exponential backoff
- Graceful degradation under load
- Performance Monitoring:
- Comprehensive metrics collection
- Processing lag monitoring
- Per-component performance tracking
This design provides a high-performance event processor capable of processing thousands of Solana transactions per second with low latency, making it suitable for Orca's requirements for real-time liquidity pool monitoring and event tracking.